Systematic Options Trading Option Greeks Strategies & Backtesting In Python
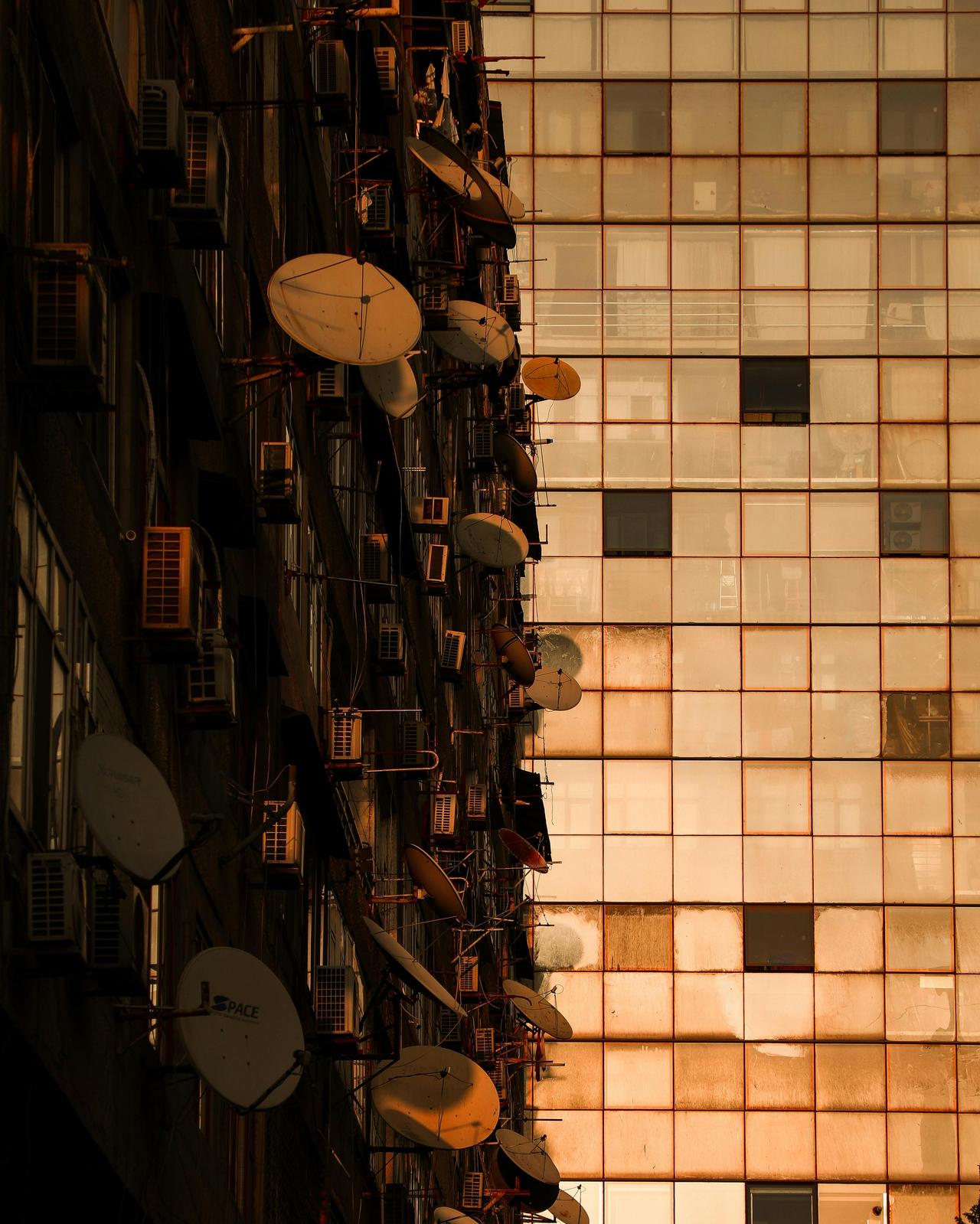
Systematic options trading involves the use of algorithmic approaches to develop and execute trading strategies based on predefined rules and quantitative models. One essential aspect of this process is understanding and applying the option Greeks, which are key metrics that measure the sensitivity of an option’s price to various factors. These Greeks—Delta, Gamma, Theta, Vega, and Rho—help traders assess the risks and potential rewards associated with different options positions.
In the realm of systematic options trading, option Greeks play a crucial role in developing strategies that can adapt to changing market conditions. For instance, Delta measures the rate of change in an option’s price relative to the underlying asset’s price movement, while Gamma indicates how Delta changes in response to underlying price fluctuations. Theta represents the time decay of an option’s value, Vega quantifies the sensitivity to changes in volatility, and Rho measures the impact of interest rate changes. By incorporating these Greeks into their trading algorithms, traders can create more robust and dynamic strategies that account for various risk factors.
Backtesting is another critical component of systematic options trading, allowing traders to evaluate the effectiveness of their strategies using historical data. By applying backtesting techniques in Python, traders can simulate how their strategies would have performed in past market conditions, helping them refine their approaches and identify potential issues before deploying them in real-time trading. Python’s rich ecosystem of libraries and tools, such as NumPy, pandas, and the backtrader framework, facilitates this process by providing powerful functionalities for data analysis, strategy development, and performance evaluation.
The integration of systematic options trading with option Greeks strategies and backtesting in Python enables traders to build sophisticated models that enhance their decision-making and risk management capabilities. By leveraging these techniques, traders can better navigate the complexities of options markets and improve their chances of achieving favorable trading outcomes.
Systematic trading leverages predefined rules and algorithms to make trading decisions based on data and statistical models. This approach aims to remove emotional bias and improve consistency in trading by automating decision-making processes. Systematic trading strategies often incorporate various financial instruments and data sources to optimize returns.
Systematic Options Trading
In systematic options trading, traders use algorithms to manage options portfolios based on predefined criteria. Key components of this strategy include understanding option greeks, developing trading strategies, and backtesting them. Option greeks, such as delta, gamma, theta, and vega, measure different aspects of options sensitivity and risk, providing valuable insights for strategy development.
Option Greeks and Their Implications
- Delta: Measures the sensitivity of the option’s price to changes in the underlying asset’s price.
- Gamma: Assesses the rate of change in delta as the underlying asset’s price changes.
- Theta: Represents the time decay of the option’s price as it approaches expiration.
- Vega: Measures the sensitivity of the option’s price to changes in volatility of the underlying asset.
These greeks help traders understand the risks and potential rewards of their options trades. By integrating these metrics into trading algorithms, traders can devise strategies that balance risk and return more effectively.
Greek | Description | Impact |
---|---|---|
Delta | Sensitivity to underlying price changes | Direct impact on option value |
Gamma | Rate of change in delta | Affects option’s price sensitivity |
Theta | Time decay | Reduces option value over time |
Vega | Sensitivity to changes in volatility | Affects option’s value with volatility |
Quote: “Systematic trading requires a thorough understanding of option greeks to effectively manage risk and optimize strategy performance. Accurate modeling and backtesting are crucial for developing successful trading algorithms.”
Backtesting Systematic Strategies
Backtesting involves applying trading algorithms to historical data to evaluate their performance. This process helps traders understand how their strategies would have performed in the past, identify potential weaknesses, and refine their approaches. Using Python for backtesting provides a flexible environment to simulate trading scenarios and analyze results.
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
# Example of backtesting a simple moving average strategy
def backtest_strategy(data, short_window, long_window):
signals = pd.DataFrame(index=data.index)
signals['price'] = data['close']
signals['short_mavg'] = data['close'].rolling(window=short_window, min_periods=1, center=False).mean()
signals['long_mavg'] = data['close'].rolling(window=long_window, min_periods=1, center=False).mean()
signals['signal'] = 0.0
signals['signal'][short_window:] = np.where(signals['short_mavg'][short_window:] > signals['long_mavg'][short_window:], 1.0, 0.0)
signals['positions'] = signals['signal'].diff()
return signals
# Load data and apply the strategy
data = pd.read_csv('historical_data.csv', index_col='date', parse_dates=True)
signals = backtest_strategy(data, short_window=40, long_window=100)
plt.figure(figsize=(12,8))
plt.plot(data['close'], label='Close Price')
plt.plot(signals['short_mavg'], label='40-Day Moving Average')
plt.plot(signals['long_mavg'], label='100-Day Moving Average')
plt.plot(signals.loc[signals.positions == 1.0].index,
signals.short_mavg[signals.positions == 1.0],
'^', markersize=10, color='g', lw=0, label='Buy Signal')
plt.plot(signals.loc[signals.positions == -1.0].index,
signals.short_mavg[signals.positions == -1.0],
'v', markersize=10, color='r', lw=0, label='Sell Signal')
plt.title('Backtest of Moving Average Strategy')
plt.legend()
plt.show()
Systematic trading, when implemented with a robust understanding of option greeks and thorough backtesting, can enhance trading efficiency and performance.
Excited by What You've Read?
There's more where that came from! Sign up now to receive personalized financial insights tailored to your interests.
Stay ahead of the curve - effortlessly.