Assignment Makes Integer From Pointer Without A Cast
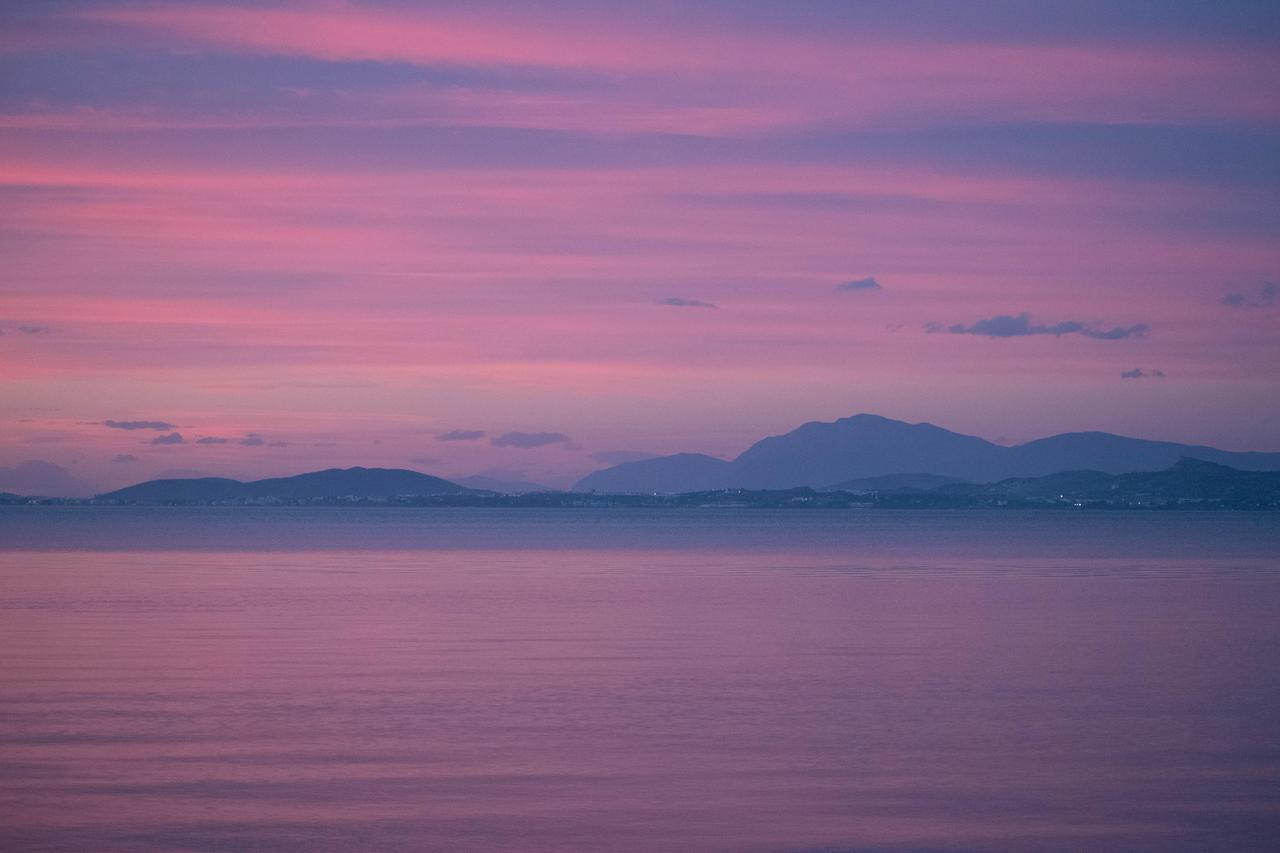
The error message “assignment makes integer from pointer without a cast” typically arises in programming languages like C and C++ when there is an attempt to assign a pointer value to an integer variable without proper type casting. This situation often occurs because pointers and integers are fundamentally different data types: pointers store memory addresses, while integers store numerical values. When a pointer is assigned directly to an integer variable, the compiler generates this error to indicate a type mismatch, which can lead to incorrect behavior or runtime errors if not properly addressed.
In a programming context, this error signifies that a programmer is trying to assign a value of type pointer (which holds a memory address) to a variable that expects an integer. For example, if you have a pointer variable int *ptr
and an integer variable int num
, attempting to do num = ptr;
would trigger the “assignment makes integer from pointer without a cast” error because ptr
is a pointer and num
is an integer.
To resolve this error, you need to perform a type cast if you intend to convert a pointer value to an integer. For instance, using num = (int)ptr;
explicitly converts the pointer ptr
to an integer value, which might be necessary if you are working with pointer arithmetic or addressing specific low-level programming scenarios. However, be cautious with type casting as it may lead to data loss or incorrect values if the pointer address cannot be accurately represented as an integer.
Conversely, if the assignment of the pointer to an integer variable was not intentional, it may indicate a logical error in the code that needs correcting. In such cases, reviewing the code to ensure that pointers and integers are used appropriately and that proper data types are maintained is crucial for maintaining code reliability and avoiding bugs.
Overall, the “assignment makes integer from pointer without a cast” error highlights the importance of understanding and managing data types correctly to ensure that assignments and operations in programming languages are performed accurately and without unintended side effects.
Assignment in programming involves assigning values to variables or data structures. It is a fundamental operation that allows for the manipulation and storage of data within a program. Depending on the programming language, assignment can involve simple value assignments, complex object assignments, or even type conversions.
Type Safety and Assignment
In strongly-typed languages, assignment requires that the types of the values being assigned are compatible with the types of the variables. For instance, assigning an integer value to a variable that expects a string could lead to errors or require explicit type casting. Proper type handling ensures that assignments are valid and prevents runtime errors or unexpected behavior.
Pointer to Integer Assignment
In some languages, particularly those that allow low-level memory manipulation like C and C++, assigning a pointer to an integer directly can lead to problems. For example, converting a pointer to an integer without casting can result in undefined behavior, as the sizes and representations of pointers and integers may differ.
Here’s an example of what this might look like in C:
int *ptr;
int num;
num = (int) ptr; // Unsafe: converting pointer to integer without proper casting
This code snippet could lead to unexpected results, as the pointer value is not correctly interpreted as an integer.
Safe Casting Practices
To avoid issues related to pointer-to-integer assignments, explicit casting and type-safe operations should be used. For instance, in C++, the reinterpret_cast
operator can be used to safely convert between pointer types and integer types when necessary, though it is generally advisable to avoid such conversions when possible.
Programming Language Differences
Different programming languages handle assignments and type conversions in various ways. In dynamically-typed languages like Python, assignment is more flexible and does not require explicit type declarations. Conversely, statically-typed languages like Java and C++ enforce strict type rules during assignment, which helps prevent type-related errors.
“In statically-typed languages, ensuring type compatibility during assignment prevents runtime errors and maintains program stability.”
Assignment and Memory Management
Memory management practices are closely related to assignment operations, particularly in languages that require manual memory management. Correctly handling memory allocation and deallocation is essential to avoid memory leaks and ensure that assignment operations do not lead to corruption or crashes.
By understanding the nuances of assignment operations and type handling, programmers can write more reliable and efficient code, preventing common pitfalls associated with improper type conversions and memory management issues.
Excited by What You've Read?
There's more where that came from! Sign up now to receive personalized financial insights tailored to your interests.
Stay ahead of the curve - effortlessly.